EXAMPLE 2
Using program loop and internal oscillator LFINTOSC
This is a continuation of the previous example, but deals with a bit more complicated problem... The idea is to make the LED diodes on the port B blink. A simple thing at first glance! It is enough to periodically change logic state on the port B. In this case, numbers 01010101 and 10101010 are selected to change in the following way:- Set binary combination 01010101 on port B;
- Remain in loop1;
- Replace existing bits combination on port B with 10101010;
- Remain in loop2; and
- Return to the step 1 and repeat the whole procedure.
You have noticed that the clock signal source has changed "on the fly". If you want to make sure of it, remove quartz crystal prior to switching the microcontroller on. What will happen? The microcontroller will not start operating because the config word loaded with the program requires the use of the crystal on switching on. If you remove the crystal later during the operation, it will not affect the microcontroller at all!
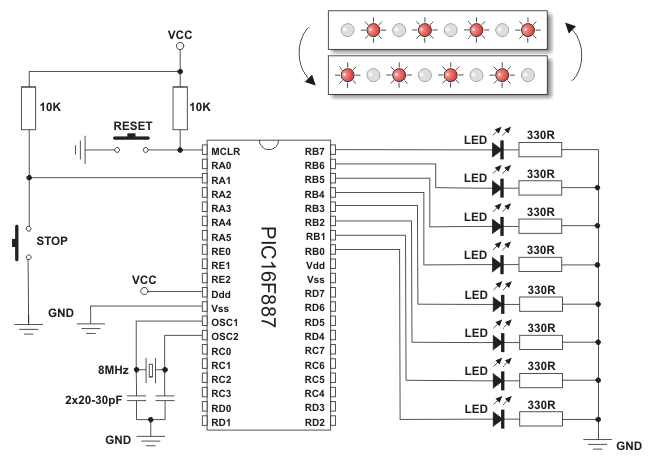
Sorce Code
;************************************************************************ ; Header ;************************************************************************ ;************* DEFINING VARIABLES *************************************** cblock 0x20 ; Block of variables starts at address 20h counter1 ; Variable "counter1" at address 20h endc ;************************************************************************ org 0x0000 ; Address of the first program instruction banksel OSCCON ; Selects memory bank containing ; register OSCCON bcf OSCCON,6 ; Selects internal oscillator LFINTOSC with bcf OSCCON,5 ; the frequency of 31KHz bcf OSCCON,4 bsf OSCCON,0 ; Microcontroller uses internal oscillator banksel TRISB ; Selects bank containing register TRISB clrf TRISB ; All port B pins are configured as outputs banksel PORTB ; Selects bank containing register PORTB loop movlw B'01010101' ; Binary number 01010101 is written to W movwf PORTB ; Number is moved to PORTB movlw h'FF' ; Number hFF is moved to W movwf counter1 ; Number is moved to variable "counter1" loop1 decfsz counter1 ; Variable "counter1" is decremented by 1 goto loop1 ; If result is 0, continue. If not, ; remain in loop1 movlw B'10101010' ; Binary number 10101010 is moved to W movwf PORTB ; Number is moved to PORTB movlw h'FF' ; Number hFF is moved to W movwf counter1 ; Number is moved to variable "counter1" loop2 decfsz counter1 ; Variable "counter1" is decremented by 1 goto loop2 ; If result is 0, continue. If not, ; remain in loop2 goto loop ; Go to label loop end ; End of program