Using Timer T2
This example describes the use of Timer T2 configured to operate in Auto-Reload mode. In this very case, LEDs are connected to port P3 while the push button used for forced timer reset (T2EX) is connected to the P1.1 pin.Program execution is similar to the previous examples. When timer ends counting, an interrupt is enabled and subroutine TIM2_ISR is executed, thus rotating a logic zero (0) in accumulator and moving the contents of accumulator to the P3 pin. At last, flags which caused an interrupt are cleared and program returns to the loop LOOP1 where it remains until a new interrupt request arrives...
If push button T2EX is pressed, timer is temporarily reset. This push button resets timer, while push button RESET resets the microcontroller.
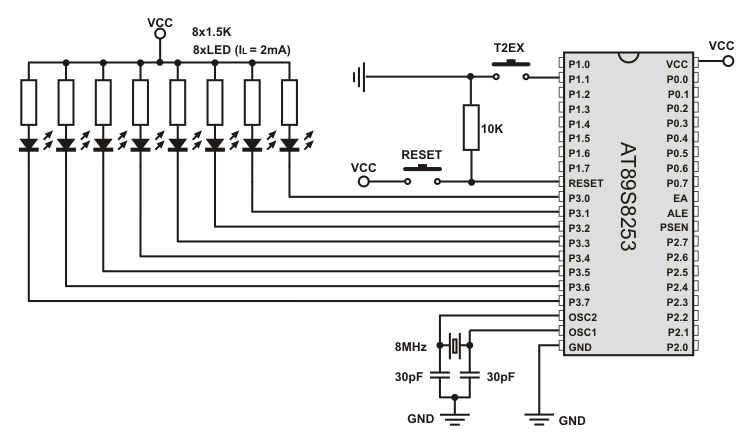
;************************************************************************ ;* PROGRAM NAME : Timer2.ASM ;* DESCRIPTION: Program rotates log. "0" on port P3. Timer2 determines ;* the speed of rotation and operates in auto-reload mode ;************************************************************************ ;BASIC DIRECTIVES $MOD53 $TITLE(TIMER2.ASM) $PAGEWIDTH(132) $DEBUG $OBJECT $NOPAGING ;DEFINITION OF VARIABLES T2MOD DATA 0C9H ;STACK DSEG AT 03FH STACK_START: DS 040H ;RESET VECTORS CSEG AT 0 JMP XRESET ; Reset vector ORG 02BH ; Timer T2 Reset vector JMP TIM2_ISR ORG 100H XRESET: MOV SP,#STACK_START ; Define Stack pointer MOV A,#0FFH MOV P3,#0FFH MOV RCAP2L,#0FH ; Prepare 16-bit auto-reload mode MOV RCAP2L,#01H CLR CAP2 ; Enable 16-bit auto-reload mod SETB EXEN2 ; Pin P1.1 reset is enabled SETB TR2 ; Enable Timer T2 MOV IE,#0A0H ; Interrupt is enabled CLR C LOOP1: SJMP LOOP1 ; Remain here TIM2_ISR: RRC A ; Rotate contents of Accumulator to the right through ; Carry flag MOV P3,A ; Move the contents of Accumulator A to PORT3 CLR TF2 ; Clear timer T2 flag TF2 CLR EXF2 ; Clear timer T2 flag EXF2 RETI ; Return from interrupt END ; End of program